Contents
Autogeneration of data fetching functions with Orval, replacing our Picture component with a new image optimisation logic, reviewing Next.js 15 updates, the power of using linter combinations. And as a little bonus, our top libraries make your project easier to maintain and develop, and save you time on initialisation.
How to use Orval for development automation
For a long time we had been creating data sampling functions manually. The implementation of Orval has helped to automate this process with full data typing of payload and response data.
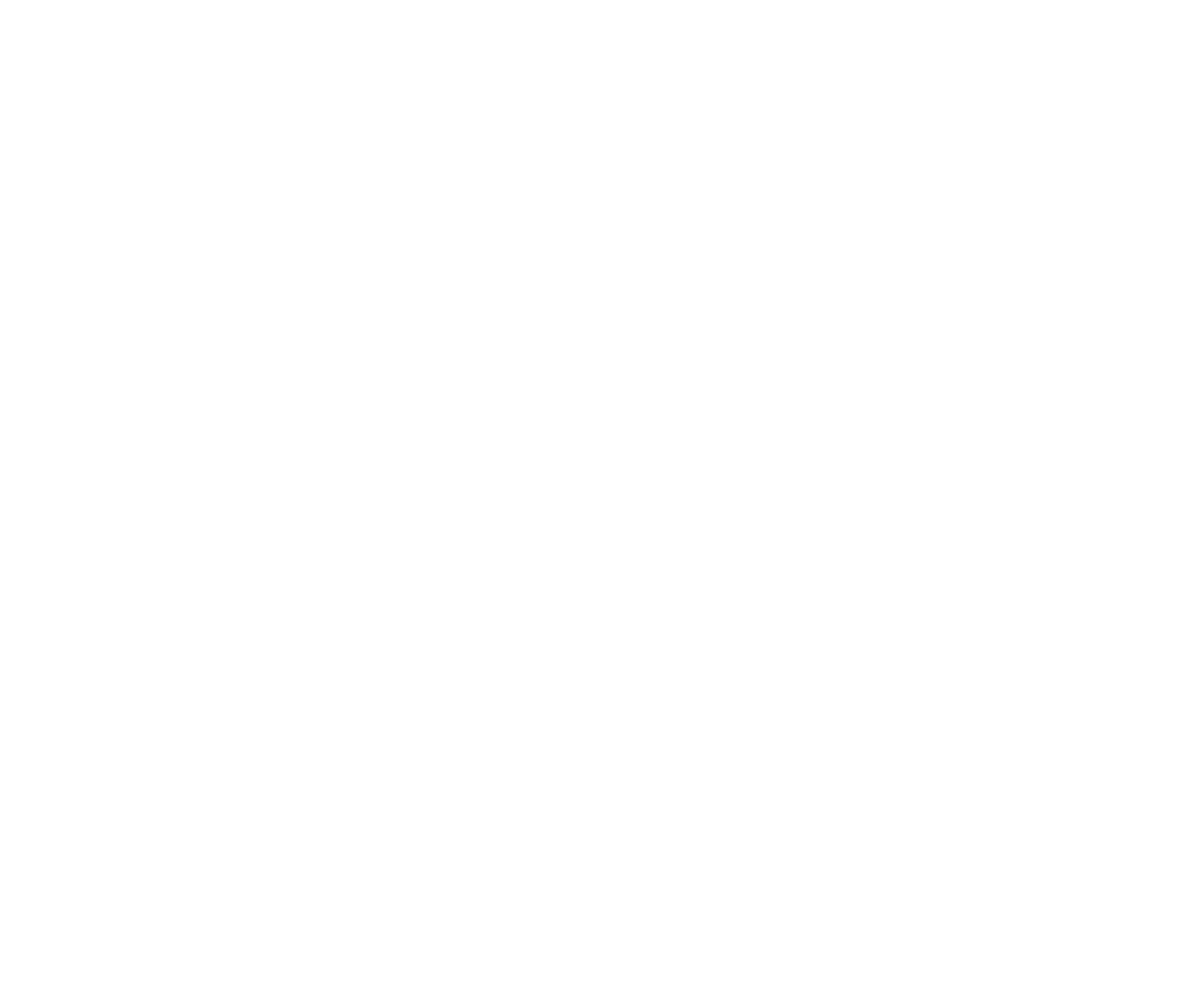
As a result, we were able to:
- Reduce development time;
- Eliminate unnecessary calls for constant clarification on projects;
- Bring documentation to a near-perfect state.
Read more about the Orval customisation experience based on a real project in this article.
Autogeneration of data fetching functions and typing with Orval
We have moved towards automation with a tool like Orval. We'll tell you how we did it, and share sample code and libraries (follow the links in the text).
The next step is to automate the creation of documentation so that back-end specialists no longer need to create it manually. They will let you know when everything is working properly.
What we have changed in the logic of working with images
So far we have written a Picture component to work with images, which includes:
- Choosing the most appropriate image size;
- Lazy loading;
- Blur version of the image displayed before loading.
The work of the component was organised in the following way: the back-end sent a structure with different image sizes to be used under certain conditions. For example, depending on the screen width, the format supported by the browser, or the number of pixels per inch. You can read more about the Picture component here.
Structure example:
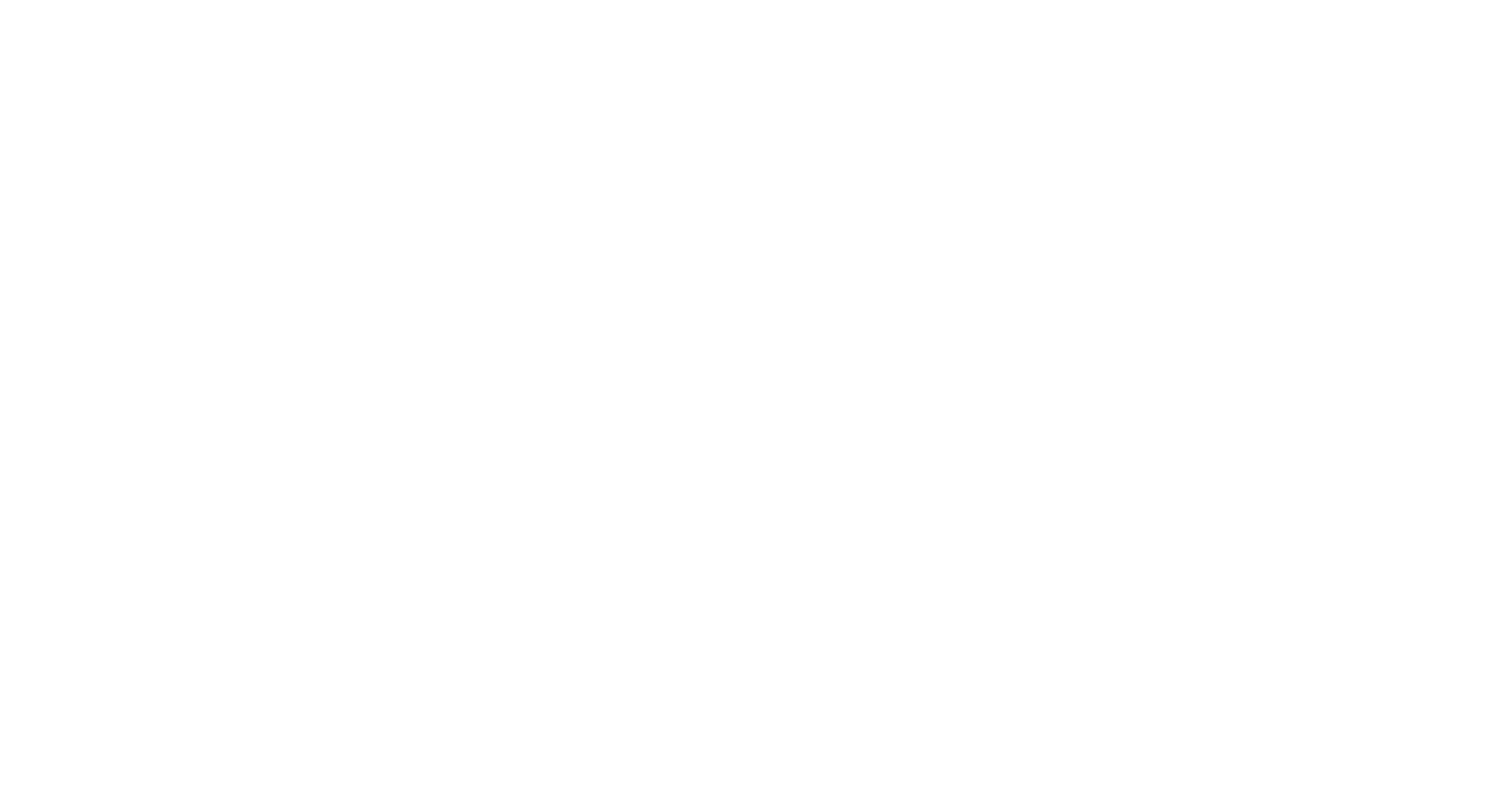
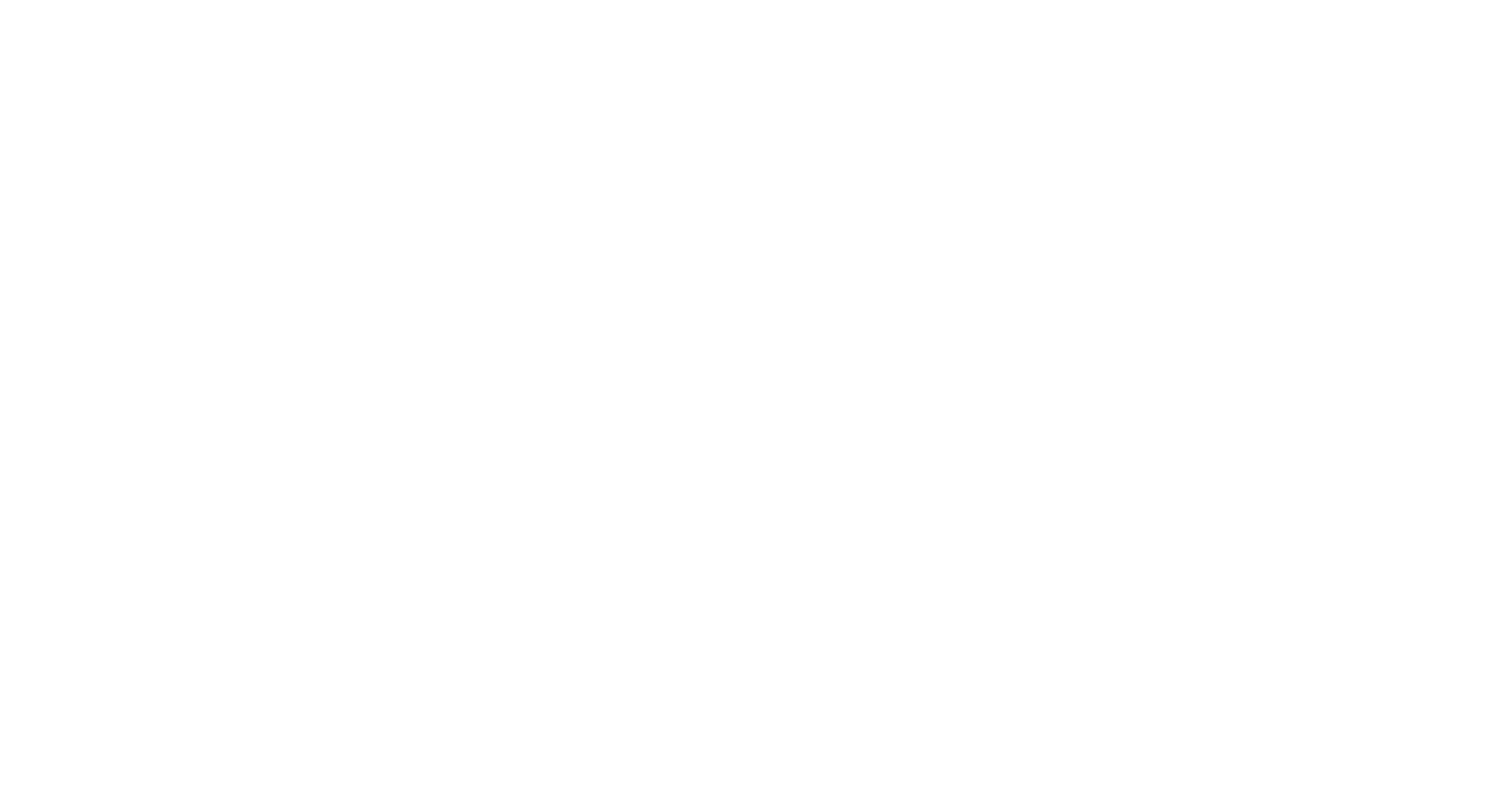
Over time, we discovered the following gaps in Picture:
- Frontend specialists needed to create dimensional images for each site component;
- Storing each entity with graphics required large structures with links to images, which increased the size of the repository and made it difficult to work with;
- The component used additional libraries to work with the canvas because of the interaction with the base83 format required for the blur version of the image.
These limitations prevented us from using the native features of the main framework we were working with. For example, image optimisation.
That's why we decided to abandon our component and fundamentally rebuild the logic of working with images.
We use Next.js on almost every project. It includes lazy loading, knows how to crop an image to the required size on its own, and then chooses the appropriate variant. The new logic assumes that we just give Next.js an image and the framework does the rest. Let's briefly describe how this process works.
To avoid loading images that have already been prepared for different devices (tablet, desktop, mobile, etc.), we will use the next/image сomponent. It uses the deviceSizes and imageSizes parameters in the Next.js configuration, which allows you to create an array of images with different widths. The browser then makes a request to the Next server (a wrapper over node.js), which compresses the image to the required size and returns it to the browser.
In the next/image you can specify the sizes parameter. This will inform the browser which image to load, depending on the width of the screen. It automatically selects the best image for the current device, improving performance. As a result, images are uploaded at the right size, rather than huge files that are then compressed on the client at a loss of quality.
To create a custom uploader instead of the default one provided by Next.js, we will use the imgproxy service. This allows us to generate links to images based on parameter titles more quickly and easily. It also optimises the download.
We are now working on step-by-step instructions for integrating the new logic into projects. So stay tuned and follow the blog for updates.
Are you ready to turn your ideas into reality? Book your free consultation
Olga V.
Business Manager
What's new in Next.js 15
At each meetup, we review updates to the major frameworks we work with.
Although Next.js 14 was recently released, there is an update for React. It was packaged in Next.js 15, although it is still RC. But even here, the Vercel developers have managed to surprise us. Let's look at the main changes.
RC (Release Candidate) – a version of the software that is almost ready for release, but may still have some bugs, and is between beta and stable.
Partial Prerendering (PPR)
For now, Next.js uses static rendering by default. Only if you are not working with dynamic functions like cookies(), headers(), query-parameters and others. Otherwise, the entire route will switch to dynamic rendering.
Partial Prerendering (PPR) allows you to combine static and dynamic components in one route. Now you can work with query-parameters without risks of damaging static rendering. As they are often used, the update will make development easier and improve application performance.
To use the new option, add the experimental.ppr key with the value ‘incremental’ to the configuration in your next.config.js file.
const nextConfig = { experimental: { ppr: 'incremental', },
};
module.exports = nextConfig;
Also add the following directive at the top of the page or layout:
export const experimental_ppr = true
experimental_ppr is applied to all childs of the route segment, including nested layouts and pages. You do not need to add it to every file, only to the top route segment.
With PPR, you can wrap any dynamic UI in a Suspense boundary. When a new request arrives, Next.js first serves the static HTML wrapper, then renders and delivers the dynamic parts in the same HTTP request.
next/after: new API for executing code after request-response streaming
This function allows arbitrary code to be executed on the server after the HTTP response has been sent to the client. You can use it to handle tasks that don't need to be completed immediately before sending a response to the user. Previously, the user had to wait for the server to respond until it had processed all the logic. Using next/after function, pending tasks can be executed in the background, allowing the server to respond immediately to the client's request.
For example, you can use this feature to send analysis actions, collect information or perform resource-intensive tasks in the background.
This option must also be included in the Next.js configuration file:
const nextConfig = { experimental: { after: true, },
};
module.exports = nextConfig;
An example of how to use this feature might be as follows:
import { unstable_after as after } from 'next/server';
export default function Layout({ children }) { after(() => { …your logic }); return <>{children}</>;
}
Other Next.js changes
- Fetch requests, GET route handlers and client navigation are no longer cached by default;
- There has been an update to the create-next-app design and a new flag to include Turbopack in local development;
- optimizePackageImports is now stable. It aims to reduce the size of the bundle and improve performance through better dependency management and compilation. The developers claim it will be cheaper than tree-shaking. This is not a new feature, for more information click here.
You can explore the full list of changes in Next.js 15 here.
What we use the linter combinations for
A heated discussion at the meetup was sparked by the question: do I need to use linter programs to clean up the code? In the end we decided what we needed. We talked about the possibility of combining eslint + styleline + prettier + husky.
Eslint
Eslint allows you to format the code according to existing rules as well as specifying your own requirements. You can use it for customisation:
- The maximum number of parameters a function can take;
- Sort imports by type (e.g. place imports of css files at the bottom by default);
- Control the array of dependencies within hooks, e.g. to remember to add data to the array depending on what logic is executed in the hook;
- Limited nesting of relative imports '../../../../components' (otherwise absolute imports must be used).
Stylelint
Helps to format styles:
- Sort properties;
- Illuminate duplicate selectors;
- Convert rgb to hex and much more.
Prettier
It also works with rule-based formatting. But it can be left out, since Eslint does exactly the same thing. Also, there are often conflicts between these linters in a combination: Eslint tries to format with one command, and Prettier does the same, but with another. So if you don't want to configure Prettier to format JSON, readme and other files, you can only use Eslint.
Husky
It helps to set up a pre-commit git hook. This means that the instructions described are executed before your commit. In our case, the Eslint and Stylelint linters will check the commit and automatically fix any problems. If there are no critical errors, the commit will be created successfully. Otherwise, you will need to manually fix the errors and re-create the commit.
This approach helps to monitor the quality of the code sent to the remote repository and to maintain an identical code base for all developers. You can also configure your IDE (e.g. VS Code) to automatically perform linter formatting in the file each time you save. And if you change the configurations, the save will occur immediately after you stop writing code.
Top libraries according to dev.family
We share our favourite selection, tried and tested by projects in our portfolio.
Orval
We talked about this above and even wrote a whole article about it. The main advantage of Orval is that the data fetching functions are generated automatically, based on a yaml-file generated by the back-end specialists. Frontenders only have to use ready-made functions. This saves time and ensures that the documentation is always up to date.
Localisation libraries
These libraries include I18-next, react-intl, next-intl. We use them everywhere, even on projects where only one language is required for development. The point is that the text is always in the same directory. When we add another language to the site, we don't have to search for the text files all over the project. It will already be in one place. All you need to do is add identical files with the new localisation and make the appropriate changes to the library configuration.
All you need to do is add identical files with the new localisation and make the appropriate changes to the library configuration. It is no longer necessary to manually search for all the places where the same text is used. Just change it in the appropriate variable in the localisation JSON file.
Figma-tokens
Right now we are testing this approach on one of our new projects. Let's briefly describe the context.
There is a plugin that allows you to export design tokens from Figma directly to a remote project repository in JSON format. This file is parsed directly into css files using certain libraries. These will contain css variables and even mixins that can be used throughout the project.
Tokens Studio (plugin) has an option to offload changes to GitLab. If everything is set up correctly, when the JSON file containing the tokens changes, the CI/CD project is rebuilt. When a project is rebuilt, all css files are generated from scratch with the changes made and applied where necessary. This means that a designer can make changes in Figma and automatically send the changes directly to the site, bypassing the front-end specialist.
In turn, the front-end specialist doesn't have to manually create all the files and variables for CSS. They will be generated automatically. This will save a lot of time at the start of the project.
That's all for now! You can read more of our articles on front-end development here. And another little bonus from us – links to all the libraries and other artefacts we've mentioned in this article:
Are you ready to turn your ideas into reality? Book your free consultation
Olga V.
Business Manager
Tags:
You may also like

Family Frontend Meetup #2
Artur Valokhin, lead frontend developer
JavaScript
08.01.202413 minutes

CSS and Next.js updates, web accessibility principles, dynamic font scaling and efficient typing for redux-thunk
Artur Valokhin, lead frontend developer
Next.js
26.09.202315 minutes

Basic REST API for sending requests to the server using Axios
Artur Valokhin, lead frontend developer
JavaScript
28.07.20239 minutes